Visually display status when looping through Google Sheets data
Show a processing status in a cell when looping through rows in Google Sheets using Google Apps Script.
Context
When working with large set of data in a Google Sheet that requires you to run computation on each row, Google Apps Script typically (automatically) batches them and then processes it — and some times, you don't get to see the end result until either the entire script / data is processed or if the script throws an error (or even times out).
Even if the script errors out, you'd most times still get to see the result for whichever row was previously processed but in a few anecdotal instances, i've seen the data not log into Google Sheets at all and so, I started forcing the script to print the result, as and when the row gets processed.
Prior reading
SpreadsheetApp.flush()
- reference here
Codebase
You can make a copy of this Google Sheet or adapt the code from below -
function loop() {
const ss = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
const baseUrl = 'https://api.hashify.net/hash/md5/hex?value=';
const [header, ...data] = ss.getDataRange().getValues();
for (let i = 0; i < data.length; i++) {
let row = data[i];
let keyword = row[header.indexOf("Keyword")];
ss.getRange(1 + i + 1, header.indexOf("Status") + 1).setValue("Fetching...");
SpreadsheetApp.flush();
let response = UrlFetchApp.fetch(baseUrl + keyword);
let hash = JSON.parse(response.getContentText()).Digest;
ss.getRange(1 + i + 1, header.indexOf("MD5 hash") + 1).setValue(hash);
ss.getRange(1 + i + 1, header.indexOf("Status") + 1).setValue("Done");
SpreadsheetApp.flush();
}
}
Demo
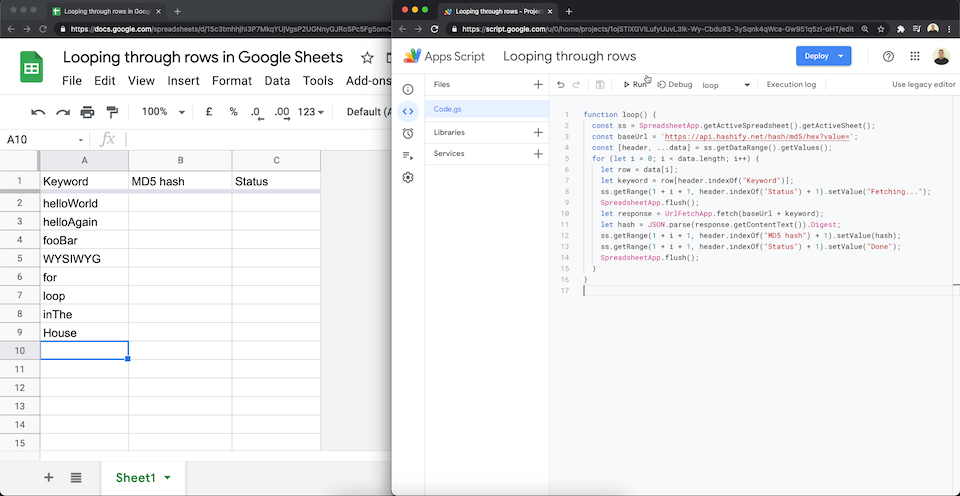