Use Firebase Dynamic Links as a URL Shortener using Apps Script
Setup a Firebase account and configure a custom domain to use it as a URL Shortener service powered by Dynamic Links and Google Apps Script.
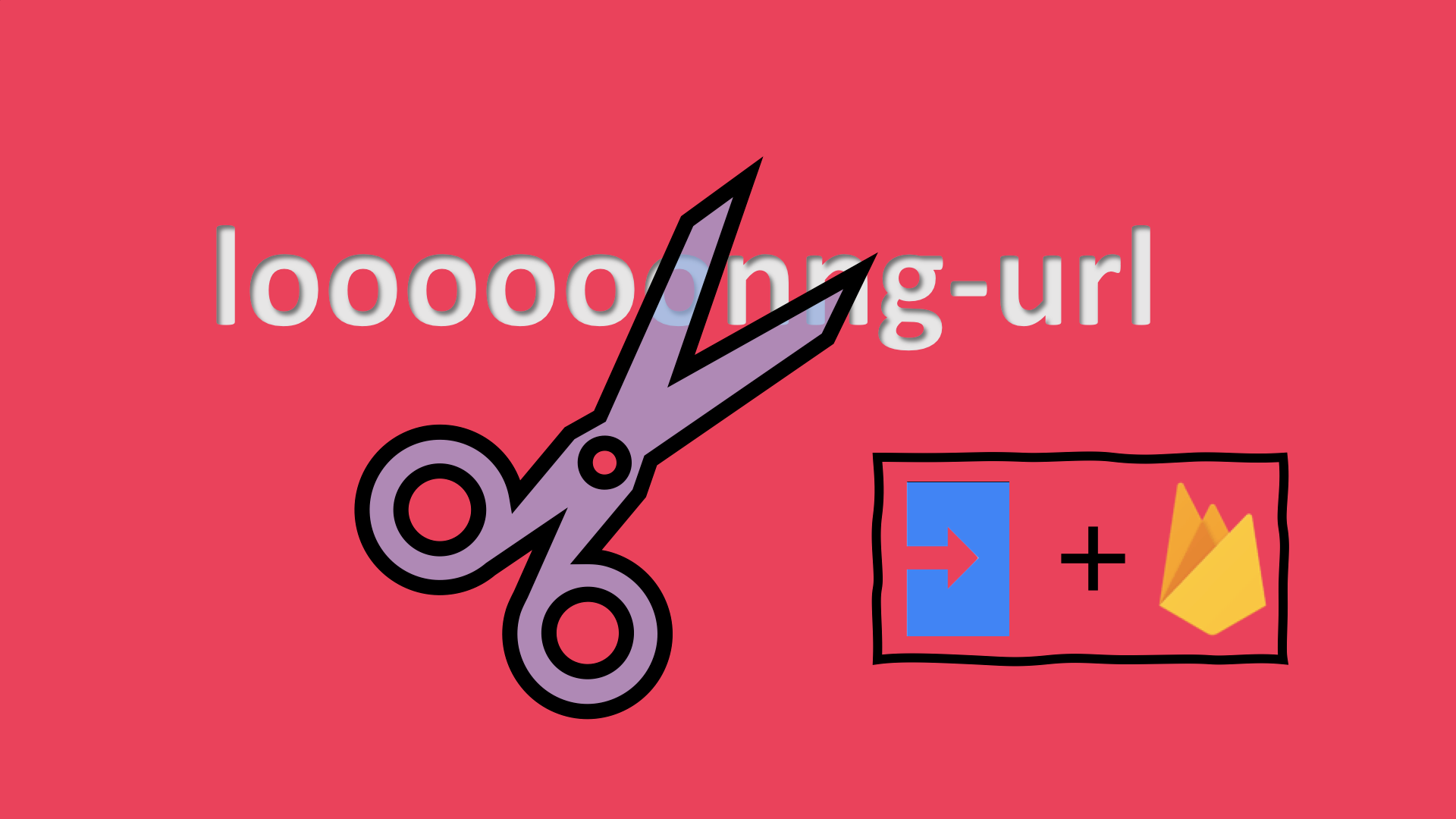
unlike the open-source url shortener service that i'd built using sheets and google apps script a few months ago, this one actually is scalable, more reliable and most importantly, not on a spreadsheet 😛
backstory
typically, when we come across firebase, we instantly think of mobile tech (among other things) and while it's usage has mostly been focussed and optimised for technologies other than that of web, we can certainly take some great advantage in building the regular products and services that we use and make them even more efficient (or so is my opinion).
there's also not much of a unique perspective on the how-to or what-to of things but since i was working on another project (involving the use of creating an add-on that interacted with firebase), thought i'd share this piece of code with some explanation that i'd written ~6 months ago, on how to get it up and running.
prior reading
here's what you need to know before diving in -
- what really is firebase (you can watch all of their videos and read all relevant documents but i don't suppose many would talk about a url shortening use-case)
- firebase > dynamic links (this is the service that we'd be using)
- the part of dynamic links that we'd be using to build a url shortener service
prerequisite
- a google cloud & firebase accounts - these are 2 separate entities and you can sign-up for their respective free tiers
- (optional) a domain name; preferably short and something that resembles your personal or professional brand; something memorable - we'd need this to configure a custom domain in firebase's dynamic links because otherwise, you'd be stuck with using their
.page.link
domains (not so pretty) - as always, 1 gmail or g suite account that allows you to make use of google apps script 😊
codebase
you can access the entire script on my github repository here but here's the gist of it -
function URLShortener() {
var body = {
"dynamicLinkInfo": {
"domainUriPrefix": "https://example.page.link",
"link": "https://example.com/?utm_source=email&utm_medium=mobile&utm_content=1234567890"
},
"suffix": {
"option": "SHORT"
}
};
var key = 'XXXXXXXXXXXXXXXXXXXXXXX'
var url = "https://firebasedynamiclinks.googleapis.com/v1/shortLinks?key=" + key;
var options = {
'method': 'POST',
"contentType": "application/json",
'payload': JSON.stringify(body),
};
var response = UrlFetchApp.fetch(url, options);
var json = response.getContentText();
var data = JSON.parse(json);
var obj = data["shortLink"];
Logger.log(obj)
}
i've set it up in a way where you only need to specify the domain name that's being used i.e. domainUriPrefix
(as configured in firebase's dynamic links service) along with the link
that you'd wish to be shortened where the predefined methodology to shorten the link is that of a short suffix that'd be handled by firebase.
you can also review all the other parameters that can be utilised while creating a dynamic link by referring to this doc here.
setup
- add a custom domain via the firebase console - you can follow through the instructions provided here
- get a web api key to run the firebase rest apis for dynamic links as indicated here
- use the aforementioned codebase & configure the required variables - mostly,
dynamicLinkInfo
,link
&key
to run the script
pro-tip: you can now use script.gs/new to launch a new google apps script project from your browser
gotchas
- in case you accidentally choose a wrong path / subdomain and want to reconfigure it, beware - you may end up with this message where you'd need to wait for a month!
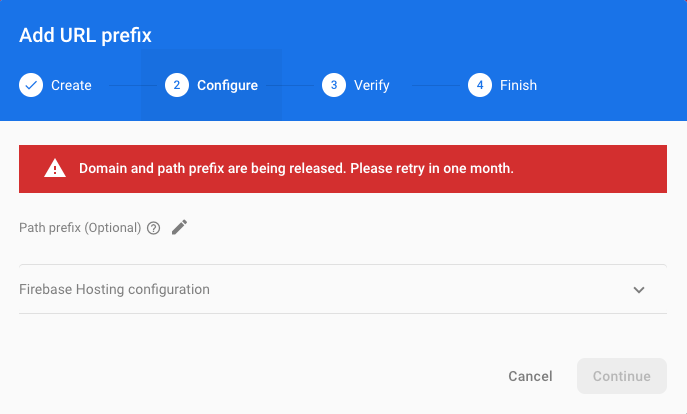
- there will be some overlap between firebase's hosting service and dynamic links - be very cautious of how you'd want the same domain behave under different circumstances and read through their documentation very carefully - here's one to look out for - priority order of hosting responses & create custom domain dynamic links (among many others)