Link to a specific conversation thread in Google Hangouts Chat
Use Google Hangouts Chat Bot services, hosted on Apps Script to identify a specific conversation thread.
It always baffles me to know how you may not be the first person to have faced a particular challenge and that tens (if not hundreds) may've already reported similar grievances.
this was oddly a scenario where after i'd devised a solution, i stumbled upon a conversation that described the exact problem and an even more precise solution.
thank you, stack exchange!
enter: getThreadID
Description
a google hangouts chat "bot" hosted on apps script, that returns a url of the conversation when invoked on a particular thread.
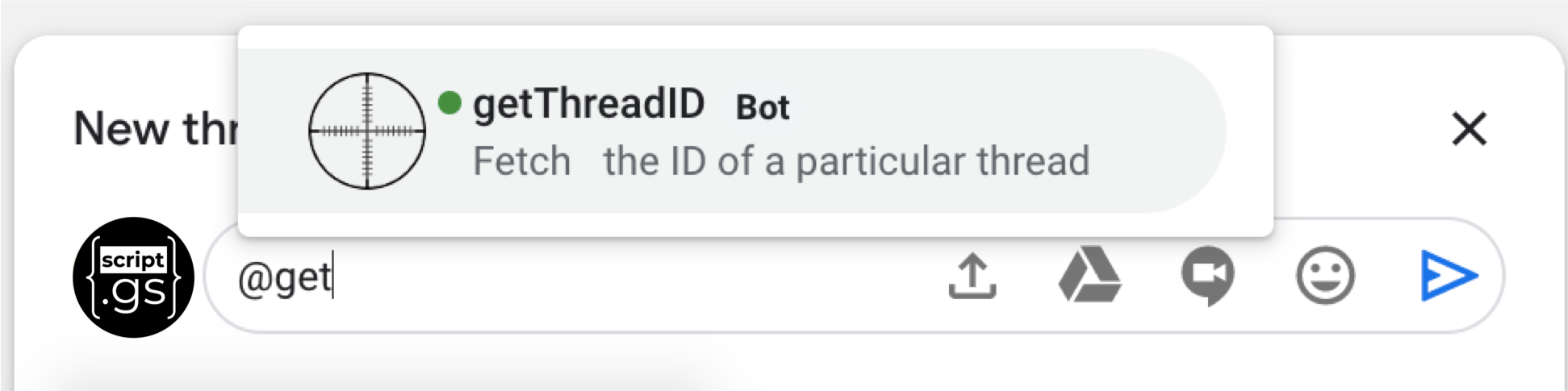
Prior reading
- google apps script bot - if you're not already familiar on how to host a hangouts chat bot on apps script, this would be an ideal place to start
- publishing bots - you'd also need to familiarise yourself with the different steps involved in actually publishing the bot, once you've adopted the code
(my two cents: just follow the instructions 🤓)
Codebase
you can access the entire script on my github repository here.
/**
* Responds to a MESSAGE event in Hangouts Chat.
*
* @param {Object} event the event object from Hangouts Chat
*/
function onMessage(event) {
var thread = event.message.thread.name;
var threadRegex = /(spaces\/)(.*)(\/threads\/)(.*)/;
var spaceID = threadRegex.exec(thread)[2]
var threadID = threadRegex.exec(thread)[4]
var message = "Thread ID: " + threadID + "\nThread URL: https://chat.google.com/room/" + spaceID + "/" + threadID;
return { "text": message };
}
/**
* Responds to an ADDED_TO_SPACE event in Hangouts Chat.
*
* @param {Object} event the event object from Hangouts Chat
*/
function onAddToSpace(event) {
var message = "";
message = "Thank you for adding me to *" + event.space.displayName + "*. \nYou can now use `@getThreadID` command to get the URL of a specific conversation.";
if (event.message) {
var thread = event.message.thread.name;
var threadRegex = /(spaces\/)(.*)(\/threads\/)(.*)/;
var spaceID = threadRegex.exec(thread)[2]
var threadID = threadRegex.exec(thread)[4]
message = "Thank you for adding me to " + event.space.displayName + "\n" + "Thread ID: " + threadID + "\nThread URL: https://chat.google.com/room/" + spaceID + "/" + threadID;
}
return { "text": message };
}
Result
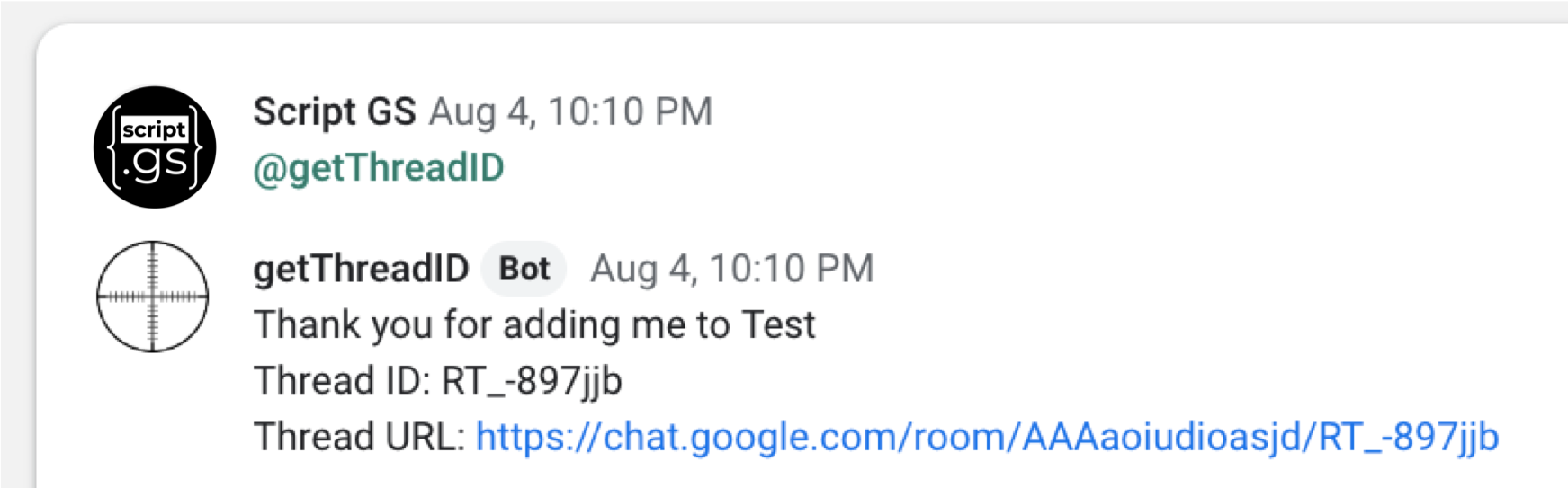