Automating attendance recorder in Google Classroom using Apps Script
Programatically create multiple questions in Google Classroom using Apps Script.
This post is going to talk about simply automating the original solution proposed by @becwest81 on collecting attendance using google classroom.
In case you haven't seen it already, the approach caught my attention when Google for Edu shared it on their twitter handle -
Learn together while apart. Check out @BecWest81's tip for recording attendance through #GoogleClassroom ↓ https://t.co/859N34dn9a
— Google for Education (@GoogleForEdu) April 4, 2020
Demo
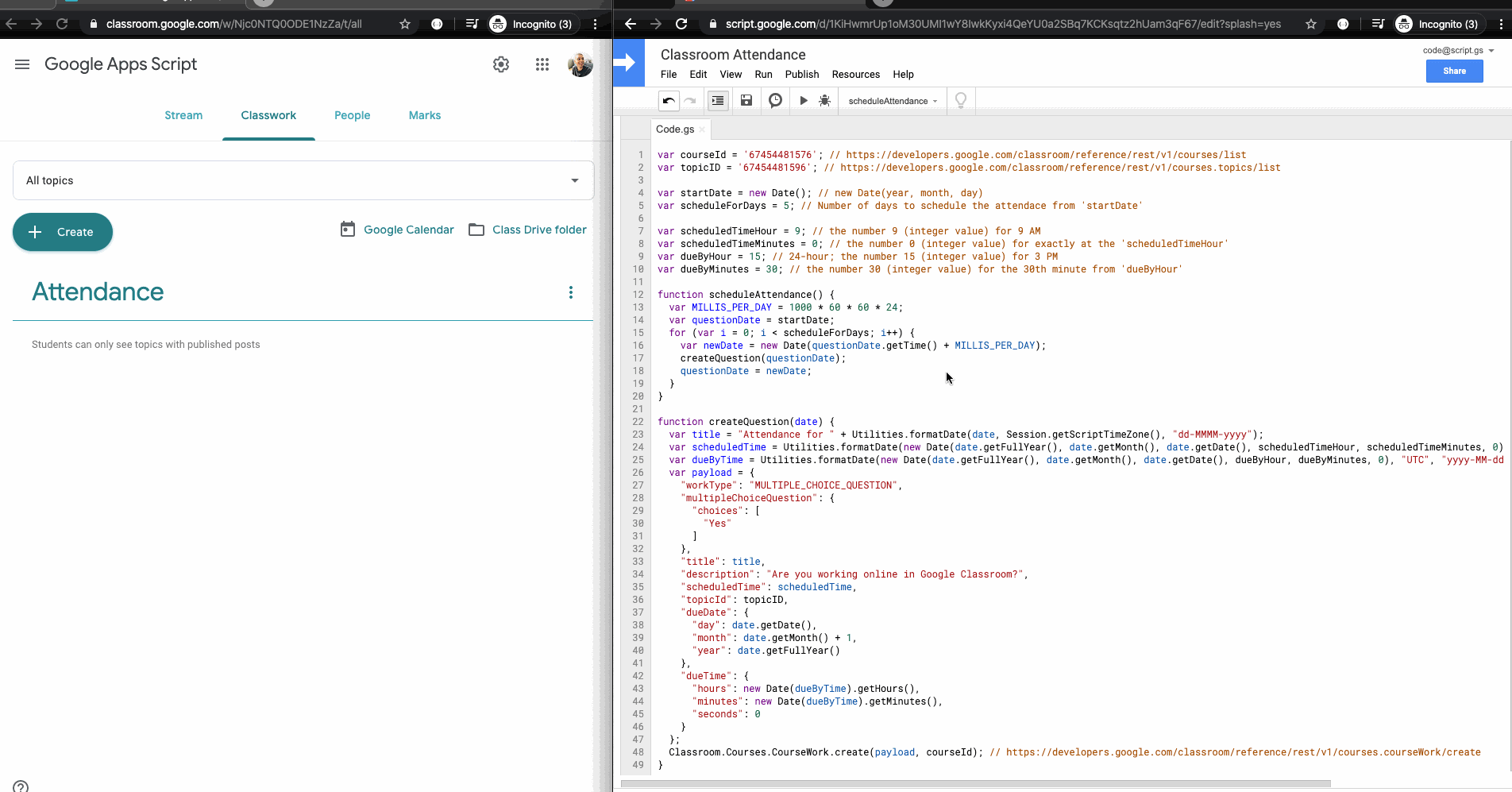
Codebase
You can access the entire script on my GitHub repository here or make a copy of my original script from this link here; however, here's the less than 50 lines of implementation for reference -
var courseId = 'XXXXXXXXXXXX'; // https://developers.google.com/classroom/reference/rest/v1/courses/list
var topicID = 'YYYYYYYYYYY'; // https://developers.google.com/classroom/reference/rest/v1/courses.topics/list
var startDate = new Date(); // new Date("dd-MMM-yyyy")
var scheduleForDays = 5; // Number of days to schedule the attendace from 'startDate'
var scheduledTimeHour = 9; // the number 9 (integer value) for 9 AM
var scheduledTimeMinutes = 0; // the number 0 (integer value) for exactly at the 'scheduledTimeHour'
var dueByHour = 15; // 24-hour; the number 15 (integer value) for 3 PM
var dueByMinutes = 30; // the number 30 (integer value) for the 30th minute from 'dueByHour'
function scheduleAttendance() {
var MILLIS_PER_DAY = 1000 * 60 * 60 * 24;
var questionDate = startDate;
for (var i = 0; i < scheduleForDays; i++) {
var newDate = new Date(questionDate.getTime() + MILLIS_PER_DAY);
createQuestion(questionDate);
questionDate = newDate;
}
}
function createQuestion(date) {
var title = "Attendance for " + Utilities.formatDate(date, Session.getScriptTimeZone(), "dd-MMMM-yyyy");
var scheduledTime = Utilities.formatDate(new Date(date.getFullYear(), date.getMonth(), date.getDate(), scheduledTimeHour, scheduledTimeMinutes, 0), "UTC", "yyyy-MM-dd'T'HH:mm:ss'Z'");
var dueByTime = Utilities.formatDate(new Date(date.getFullYear(), date.getMonth(), date.getDate(), dueByHour, dueByMinutes, 0), "UTC", "yyyy-MM-dd HH:mm:ss");
var payload = {
"workType": "MULTIPLE_CHOICE_QUESTION",
"multipleChoiceQuestion": {
"choices": [
"Yes"
]
},
"title": title,
"description": "Are you working online in Google Classroom?",
"scheduledTime": scheduledTime,
"topicId": topicID,
"dueDate": {
"day": date.getDate(),
"month": date.getMonth() + 1,
"year": date.getFullYear()
},
"dueTime": {
"hours": new Date(dueByTime).getHours(),
"minutes": new Date(dueByTime).getMinutes(),
"seconds": 0
}
};
Classroom.Courses.CourseWork.create(payload, courseId); // https://developers.google.com/classroom/reference/rest/v1/courses.courseWork/create
}
Setup
- get the
courseId
andtopicID
by visiting the following resources and trying out the API explorer -- to get the course id: https://developers.google.com/classroom/reference/rest/v1/courses/list
- to get the topic id: https://developers.google.com/classroom/reference/rest/v1/courses.topics/list
- note that you'll need the course id from the first link to be able to get the topic id from the second
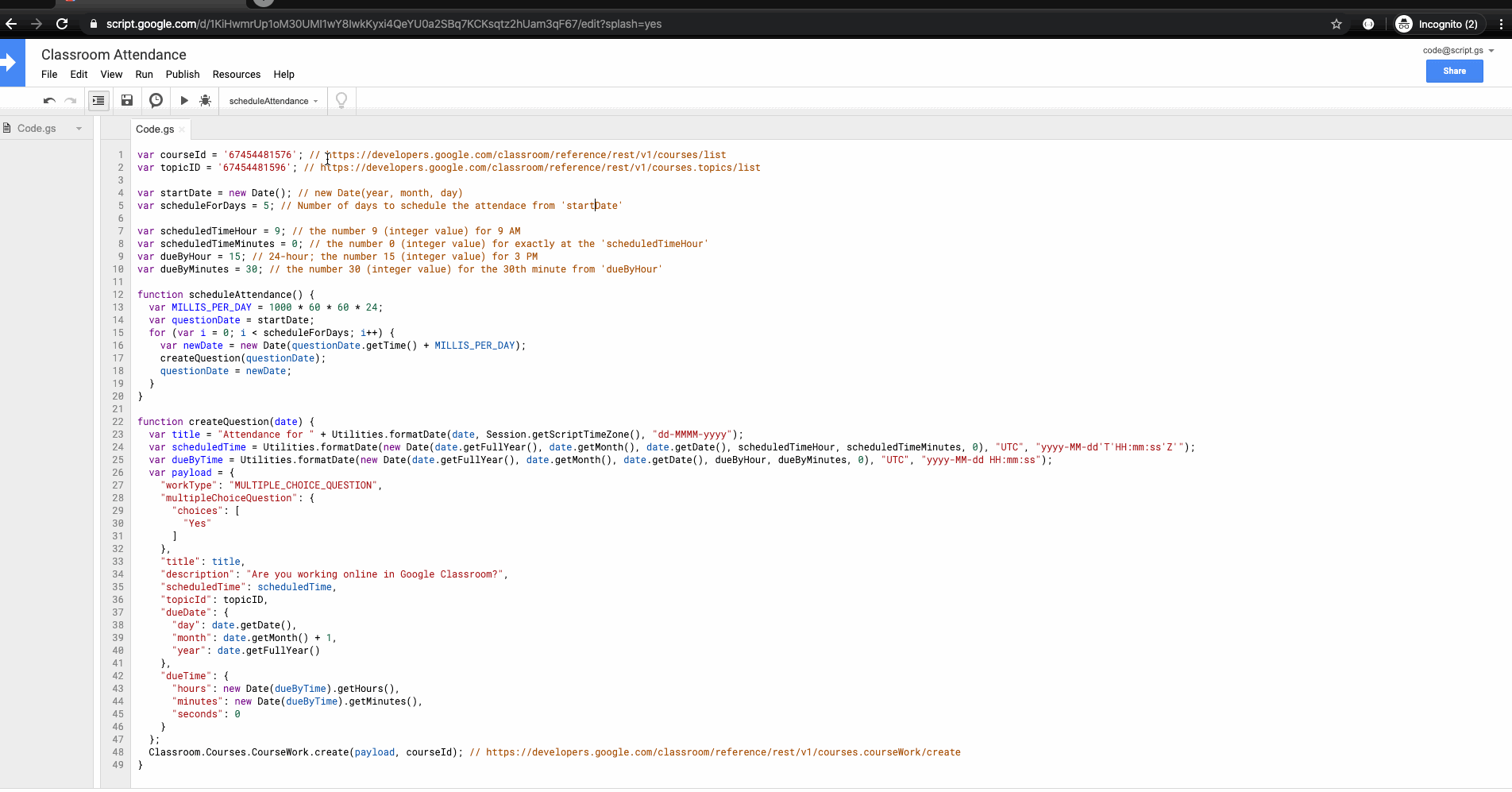
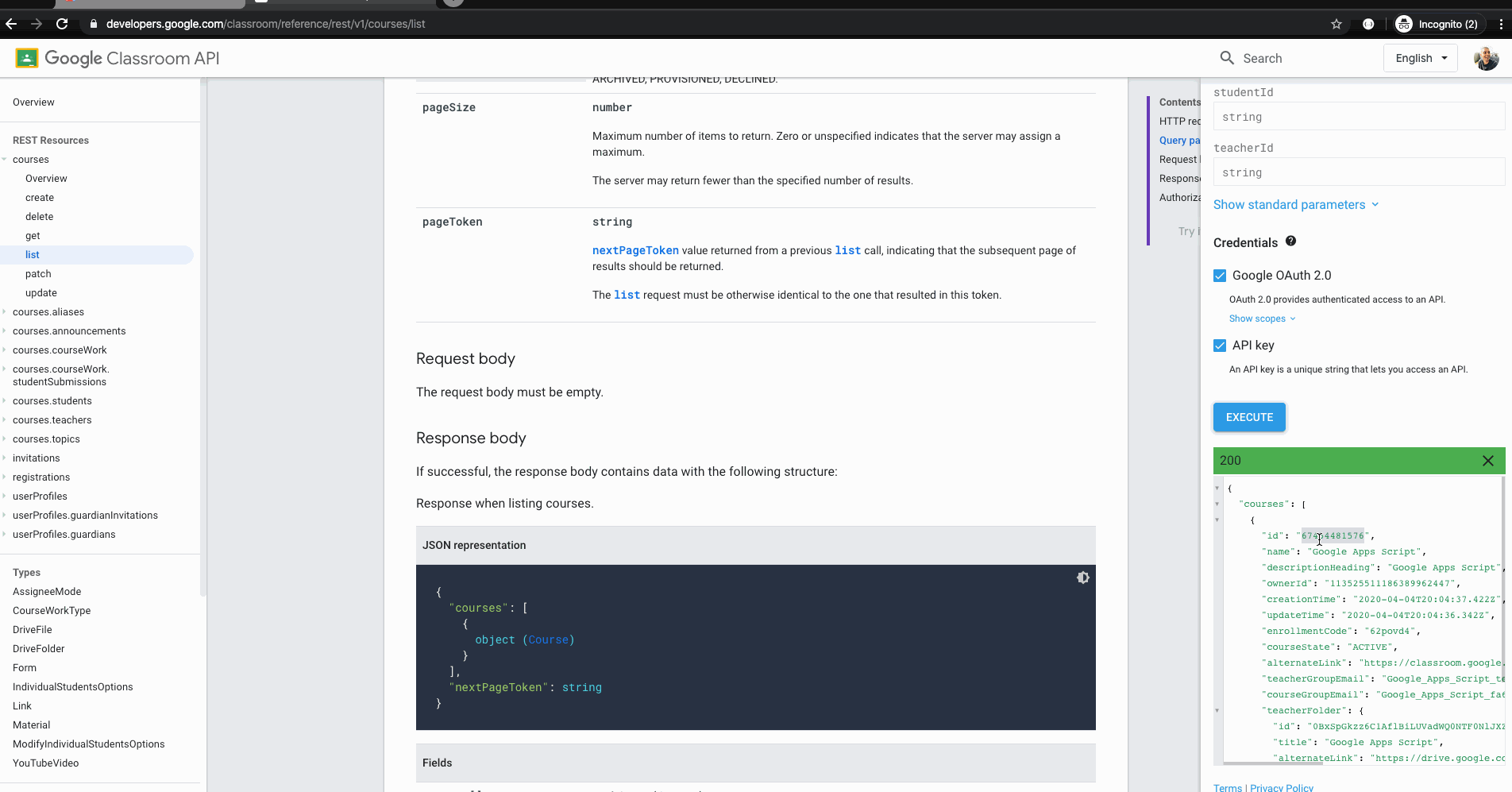
- replace the course and topic ids in the script to the ones you get from step 1
- the classroom service is an advanced google service in apps script and as such, will require you to first enable it before use
- configure the following variables before running the script -
startDate
: in case you wish the script to start right from today, then leave it as is and modify the next variables accordingly- note that the start date needs to be greater than the 'now' of whenever you intend to trigger the script i.e. the apis cannot create/schedule for an older/elapsed date
- if you wish to start scheduling from a particular day, modify the
new Date()
tonew Date(dd-MMM-yyyy)
wheredd-MMM-yyyy
would be the date in 2 digits (dd), month in letters but only the first 3 characters of any month (ex:Apr
) and year in full (yyyy)
scheduleForDays
: this is to know for how many days starting fromstartDate
would you want to create/schedule the attendance questionaire forscheduledTimeHour
: what hour of the time would you want to schedule it forscheduledTimeMinutes
: you're free to specify a minute against the scheduled hour
dueByHour
: a due-by hour for when you'd like to stop receiving responses for that daydueByMinutes
: similarly a due-by minutes should it be anything other than 0
- finally, just run the
scheduleAttendance
function to trigger the automation
In case you find any difficulties with configuring the same, please feel free to reach out to me on code@script.gs
or you could also connect with me over at Twitter.