Access Notion API with 'OAuth2 for Apps Script' library
Insights from a first-time Apps Script OAuth2 library user when connecting to the Notion API.
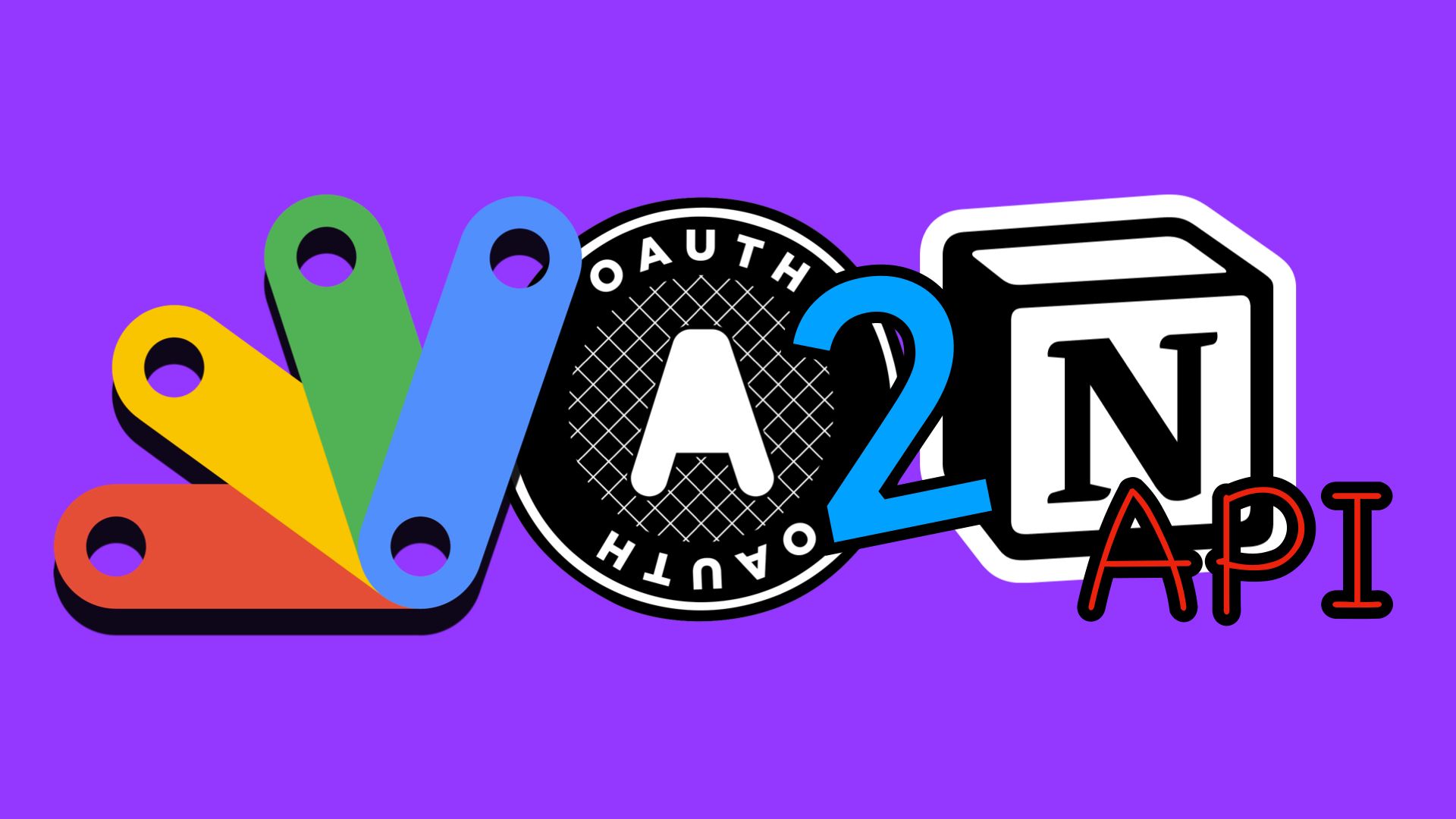
If you're a first time Google Apps Script OAuth2 library user like me then this video might be of help 🤓
In this video I take the Notion API (beta) as an example to demonstrate how to go about setting up the OAuth2 library inside your Apps Script project and going about configuring the right pieces of code.
Codebase
You can access the entire script on my GitHub repository here.
var CLIENT_ID = '...';
var CLIENT_SECRET = '...';
/**
* Authorizes and makes a request to the Notion API - https://developers.notion.com/reference/intro
*/
function run() {
var service = getService();
if (service.hasAccess()) {
var url = 'https://api.notion.com/v1/search'; // refer https://developers.notion.com/reference/post-search
var options = {
'method': 'post',
'muteHttpExceptions': true,
'headers': {
'Notion-Version': '2021-05-13', // refer https://developers.notion.com/reference/versioning
'Authorization': 'Bearer ' + service.getAccessToken()
}
};
var response = UrlFetchApp.fetch(url, options);
var result = JSON.parse(response.getContentText());
Logger.log(JSON.stringify(result, null, 2));
} else {
var authorizationUrl = service.getAuthorizationUrl();
Logger.log('Open the following URL and re-run the script: %s', authorizationUrl);
}
}
/**
* Reset the authorization state, so that it can be re-tested.
*/
function reset() {
getService().reset();
}
/**
* Configures the service.
*/
function getService() {
return OAuth2.createService('Notion')
// Set the endpoint URLs.
.setAuthorizationBaseUrl('https://api.notion.com/v1/oauth/authorize')
.setTokenUrl('https://api.notion.com/v1/oauth/token')
// Set the client ID and secret.
.setClientId(CLIENT_ID)
.setClientSecret(CLIENT_SECRET)
// Set the name of the callback function that should be invoked to
// complete the OAuth flow.
.setCallbackFunction('authCallback')
// Set the property store where authorized tokens should be persisted.
.setPropertyStore(PropertiesService.getUserProperties())
.setTokenHeaders({
'Authorization': 'Basic ' + Utilities.base64Encode(CLIENT_ID + ':' + CLIENT_SECRET)
});
}
/**
* Handles the OAuth callback.
*/
function authCallback(request) {
var service = getService();
var authorized = service.handleCallback(request);
if (authorized) {
return HtmlService.createHtmlOutput('Success!');
} else {
return HtmlService.createHtmlOutput('Denied.');
}
}
/**
* Logs the redict URI to register.
*/
function logRedirectUri() {
Logger.log(OAuth2.getRedirectUri());
}